10 Java Hacks That Most Junior Developers Don’t Know!
There are two types of developers - Good developers and Smart Developers. Today I will discuss 10 Java tips and tricks that will help to become Smart Developers. So let’s start with any further delays.
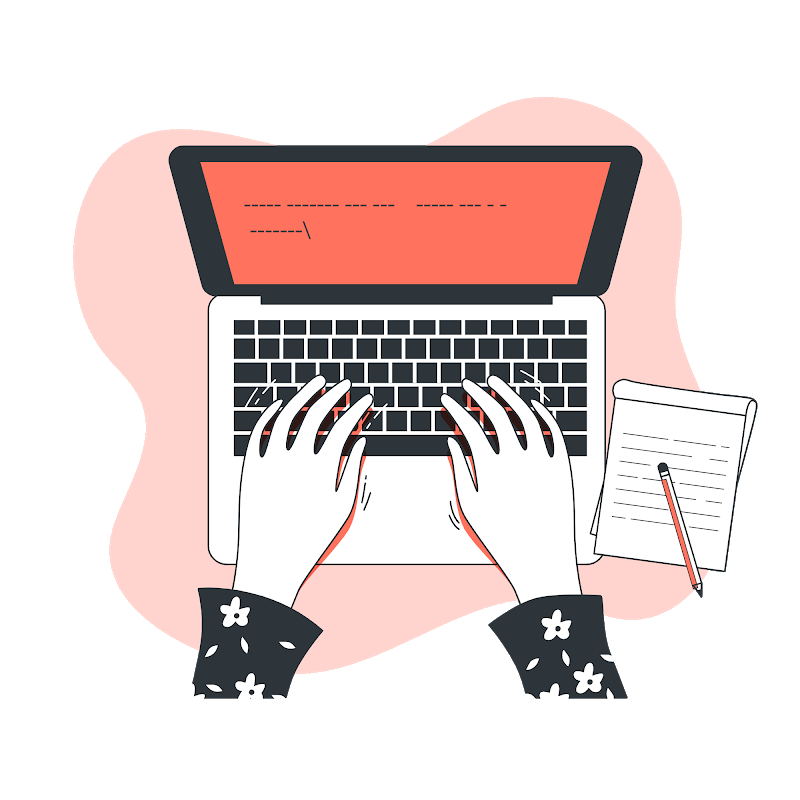
1) Different ways to write the main() function
From your absolute first day with Java, you might be writing below code,
public class Example {
public static void main(String [ ] args){
System.out.println(“Hello World”);
}
}
And that’s quite legit, right? Now let me show you different ways of writing main(),
Way One:
public class Example {
public static void main(String . . . args){
System.out.println(“Hello World”);
}
}
You can replace those [ ] brackets with just simple three dots “. . .” And still, your code will work absolutely fine.
Way Two:
public class Example {
static public void main(String . . . args){
System.out.println(“Hello World”);
}
}
Also, you can shuffle the statement public static void main() and write as static public void main() and so on, it will work fine!
2) Easy Swapping
You might surely have come across this problem, Swapping numbers using Java. And you might have tried the old school method of using the temporary variable or the one method shown below,
class Example {
public static void main(String a[]) {
int x = 10;
int y = 5;
x = x + y;
y = x - y;
x = x - y;
System.out.println("After swaping:"+ " x = " + x + ", y = " + y);
}
}
Now, let me show you the smart way,
class Example {
public static void main(String a[]) {
int x = 10;
int y = 5;
y= x+y - (x=y);
System.out.println("After swaping:"+ " x = " + x + ", y = " + y)
}
}
3) Java code without main() function
You might be thinking about, what I am saying, Java code without main(). From the first day, we have seen that Java programs cannot run without the main() function. But here’s a trick using a static block. Check this out,
{
static
{
System.out.println("Hello World!");
System.exit(0); // prevents “main method not found” error
}
}
Exciting right?
4) Measuring Program Runtime
Want to measure the time required by the program to run? Or there are many applications requiring a very precise time measurement. For this purpose, Java provides static methods in System class:
currentTimeMillis(): Returns current time in MilliSeconds since Epoch Time, in Long.
long startTime = System.currentTimeMillis();
long estimatedTime = System.currentTimeMillis() - startTime;
nanoTime(): Returns the current value of the most precise available system timer, in nanoseconds, in long. nanoTime() is meant for measuring relative time intervals instead of providing absolute timing.
long startTime = System.nanoTime();
long estimatedTime = System.nanoTime() - startTime;
5) Checking for odd numbers
Have a look at the lines of code below and determine if they can be used to precisely identify if a given number is Odd?
public boolean oddOrNot(int num) {
return num % 2 == 1;
}
These lines seem correct but they will return incorrect results one of every four times. Considering a negative Odd number, the remainder of the division with 2 will not be 1. So, the returned result will be false which is incorrect!
Now check this out!
public boolean oddOrNot(int num) {
return (num & 1) != 0;
}
Using this code, not only is the problem of negative odd numbers solved, but this code is also highly optimized. Since Arithmetic and Logical operations are much faster compared to division and multiplication, the results are achieved faster so in the second snippet.
6) Searching for strings in Java
This might not be a so-called trick, but it is something that you should know as a Java developer. Java offers a Library method called indexOf(). This method is used with String Object and it returns the position of the index of desired string. If the string is not found then -1 is returned.
public class Example {
public static void main(String[] args) {
String myString = "Hello String!";
if(myString.indexOf("String") == -1) {
System.out.println("String not Found!");
}
else {
System.out.println("String found at: " + myString.indexOf("String"));
}
}
}
7) Smart Decision Making
When we talk about decision making, the first thing comes to our mind is If-else. And that is the mast easy way of making decisions in Java. Check this below example of if-else,
class Example{
public static void main(String [ ] main){
int n1 = 5;
int n2 = 10;
if(n1>n2){
System.out.println(“n1 is greater”);
}
else{
System.out.println(“n2 is greater”);
}
}
}
Phwee…
That’s an old school and quite big! Not check below example using Ternary operator,
class Ternary {
public static void main(String[] args)
{
int n1 = 5;
int n2 = 10;
int max;
max = (n1 > n2) ? n1 : n2;
System.out.println("Maximum is = " + max);
}
}
Awesome right? That’s why I call it, Smart Decision Making!
8) Using Stack Trace
Programming and Errors! It’s Quite a better love story than Twilight! Jokes apart,
Catching bugs is perhaps the most time-consuming part of the Java development process.
The stack trace allows you to track exactly where the project threw an exception.
Check this out!
import java.io.*;
Exception e = …;
java.io.StringWriter sw = new java.io.StringWriter();
e.printStackTrace(new java.io.PrintWriter(sw));
String trace = sw.getBuffer().toString();
9) User Input using System.in.read()
User input is one of the important aspects of any application. Talking about Java, You might be familiar with the below to methods to take user input,
- Using Scanner Class
- Using BufferedReader
Today I will show you the one more exciting way of taking user input using
System.in.read(). Only thing is, it reads one byte at a time!
Check out the below example,
import java.io.IOException;
class ExamTest{
public static void main(String args[]) throws IOException{
int sn=System.in.read();
System.out.println(sn);
}
}
10) Use Strings Carefully
If two Strings are concatenated using the “+” operator in a “for” loop, then it creates a new String Object, every time. This causes wastage of memory and increases performance time. Also, while instantiating a String Object, constructors should be avoided and instantiation should happen directly.
For example:
//Slower Instantiation
String bad = new String("Yet another string object");
//Faster Instantiation
String good = "Yet another string object"
Thus, know this Java hacks and impress your senior developers and your Boss!
Want to learn Java? Check out Programming Hero to learn coding in a
fun way!