Switching from Java to Kotlin in 5 Minutes
Java vs Kotlin has been one of the most common debates over the past year. As an android app developer, you know that Kotlin seems to be the future. Yes, I know it is tough to leave a programming language like Java that we have been using since the start for android development, but at some point, we have to! It’s just another bitter truth. Well, in this article, I will help you switch from Java to Kotlin in 5 minutes.
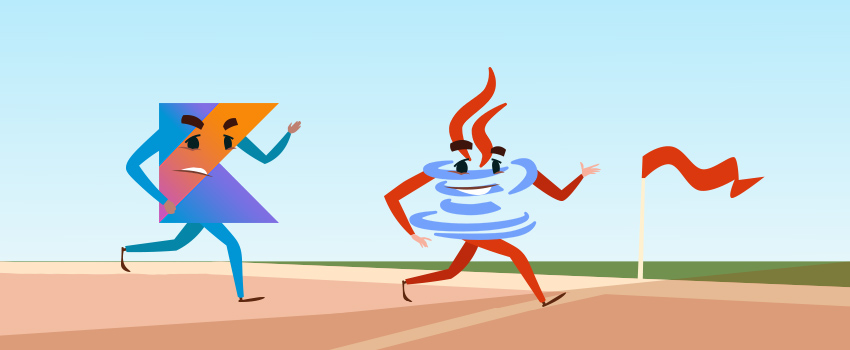
But before that, let’s have a look at Kotlin, and simple 3 reasons to switch from Java to Kotlin -
What is Kotlin?
Developed by Jetbrains, Kotlin is a cross-platform, statically typed, general-purpose programming language with type inference. Similar to Java, Kotlin has become a top choice for developing Android applications. Kotlin is designed to interoperate fully with Java, and the JVM version of Kotlin's standard library depends on the Java Class Library, but type inference allows its syntax to be more concise.
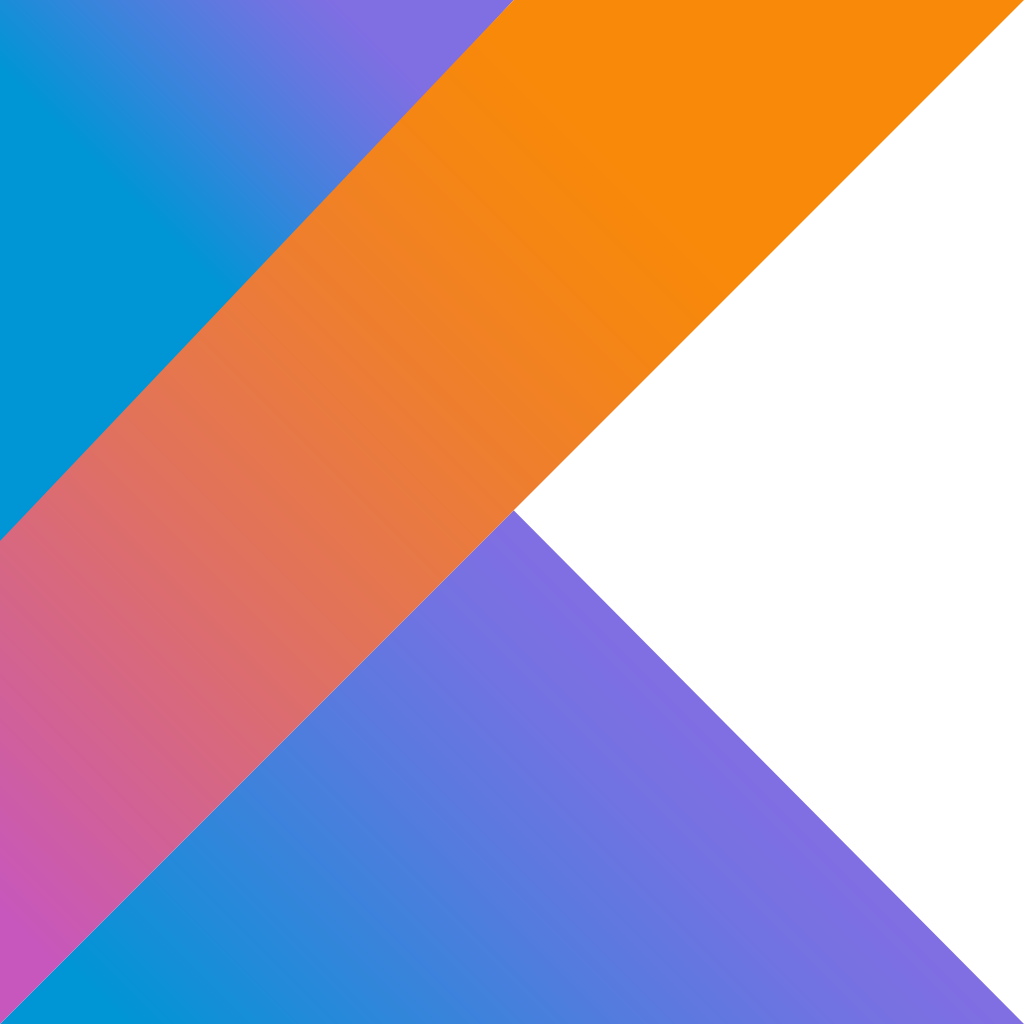
Well, Kotlin is yet another language like Java but has managed to gain quite more attraction and love by the developers. Android app developers around the globe have started moving towards Kotlin. Below are three simple reasons why you should switch to Kotlin right away,
Official Language for Android Development
Recently, Google announced Kotlin as the official language for Android Development. That came as a bit of a surprise, given that Java had long been the preferred language for Android app development, but few announcements at that year’s I/O got more applause. Over the course of the last two years, Kotlin’s popularity has only increased. More than 50% of professional Android developers now use the language to develop their apps. Sounds convincing, right?
New Libraries are in Kotlin
Well, with the rise of Kotlin and its increasing popularity, Kotlin is one of the most preferred languages for Android developers. All the new libraries that come up are generally written in Kotlin. Ofcourse, they support Java and have a Java version as well. But, Kotlin has now become the core choice and the default language for the development of various libraries and plugins.
Less Code and Concise Syntax
As a developer, you would surely prefer something that gets done in a few lines of code. Well, this is one of the main reasons for the rise of Kotlin. Kotlin takes around 30% less code than Java to get the things code. Amazing right? Even Kotlin’s syntax is far much simpler and concise. Thus, Kotlin provides you with clean and concise code with fewer lines
And the list goes on if we start comparing Java and Kotlin. Well, that might be a discussion for some other time, but these three reasons are strong and convincing itself.
Now let’s start with Kotlin and learn how to switch for Java to Kotlin.
Switching from Java to Kotlin
Well, we will see some of the most common programming syntax and concepts and learn how to write them using Kotlin.
Print Statement - Hello World
Well, there is not much difference here while printing, but Kotlin syntax is much more readable and English like. Even it has a bit less syntactic sugar that makes you a bit more comfortable with the language. Let’s have a look,
Java -
public class Demo{
public static void main(String [ ] args){
System.out.println(“Hello World”);
}
}
Kotlin -
fun main() {
println("Hello, World!")
}
2) Variable Deceleration
Well, variable deceleration is pretty simple in Kotlin. In Kotlin, we don’t need to mention the data type explicitly, it automatically determines it. Also, Kotlin uses two keywords namely, val and var. The val keyword will make your variable immutable, just like the final keyword in Java and the var keyword makes a variable mutable. One more thing to note here in Kotlin is, it does not use semicolons. Oh, that’s a big relief😇
Let’s have a look,
Java -
int number = 5;
final String name = “John”;
Kotlin -
var number = 5
val name = “John”
3) Loops
There are no traditional loops in Kotlin unlike Java and other languages.
In Kotlin, loops are used to iterate through ranges, arrays, maps, and so on (anything that provides an iterator). Just like in Python.
Kotlin provides a concept of range. The range goes from one number to another.
Below is how it looks,
Java -
for(int i = 0; i <5; i++){
System.out.println(“ ”+ i);
}
Kotlin -
for(i in 1..5){
println(i)
}
4) The Switch Case
Well, Kotlin does not have a switch statement. Kotlin provides a when statement instead. The when construct in Kotlin can be thought of as a replacement for Java switch Statement. It evaluates a section of code among many alternatives.
For Example,
Java -
int x = 2;
switch(x){
case 1:
System.out.println(“1”);
break;
case 2:
System.out.println(“2”);
break;
default:
System.out.println(“None”);
}
Kotlin -
val x = 2
when(x) {
1 -> println(“1”)
2 -> println(“2”)
else -> println(“None”)
}
Much simple and better, right?
5) Functions
Defining functions in Kotlin is a bit different. To define a function in Kotlin, a fun keyword is used. Then comes the name of the function. In Kotlin, arguments are separated using commas. Also, the type of formal argument must be explicitly typed. Also, we can optionally, write the data type of the returned value. Below is how it looks,
Java -
int add(int a, int b){
int sum = a + b;
return sum;
}
Kotlin -
fun add(n1: Int , n2: Int): Int {
val sum = n1 + n2
return sum
}
6) Classes and Objects
Well, the syntax to define a class in Kotlin is pretty similar. The difference is when we define a new object. In Kotlin, we do not need the ‘new’ keyword to create a new object.
Java -
class User() {
----
}
User newUser = new User();
Kotlin -
class User() {
----
}
var newUser = User()
7) Inheritance in Kotlin
Inheritance is one of the important concepts of programming. By default, classes in Kotlin are final. If you are familiar with Java, you know that a final class cannot be subclassed. By using the open annotation on a class, the compiler allows you to derive new classes from it. Also, there is no ‘extends’ keyword in Kotlin, we use a simple colon.
Java -
class Demo1(){
-----
}
class Demo2 extends Demo1(){
---
}
Kotlin -
open class Demo1(){
----
}
class Demo2: Demo1(){
---
}
8) Getters and Setters - POJO Objects
Getter and Setter are some of the important concepts when it comes to android development. It is pretty simple in Kotlin though. In Kotlin we don’t define getters and setters, they are automatically generated behind the scenes. The primary constructor is also generated behind the scenes. Thus, it makes our job pretty simple. Let’s have a look at how it works,
Java -
class Person{
private String name;
private int age;
public Person(String name, int age){
this.name=name;
this.age=age;
}
public void setName(String name){
this.name = name;
}
public String getName(){
return name;
}
public void setAge(int age){
this.age = age;
}
public int getAge(){
return age;
}
}
Kotlin -
data class Person{
val name: String;
val age: int;
}
Well, that’s why developers love Kotlin! It is not possible to cover everything in an article, but all the above are the generally used concepts in Android and will help you switch from Java to Kotlin. Want to dive deep in Java and Kotlin? And want to learn Android development? Try Programming Hero , a fun way to learn to code.