20 Python Tips and Tricks for New Python Developers
There are two types of developers - Good developers and Smart Developers. Today I will discuss 20 Python tips and Tricks that will help to become Smart Developers. So let’s start with any further delays.
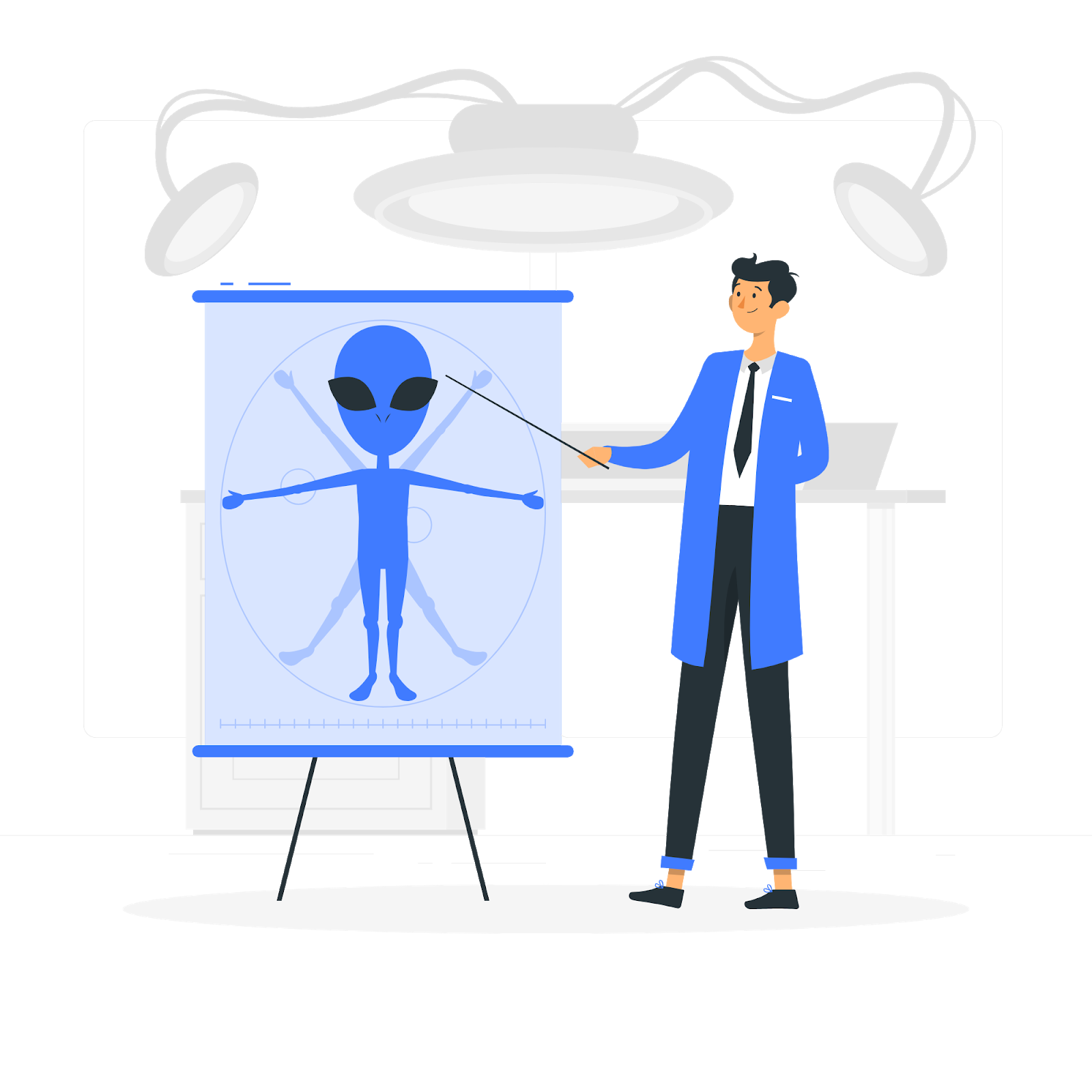
1) Swapping numbers :
Swapping is an important concept when it comes to data structures and algorithms. Let’s see how we can swap numbers in Python,
Normal Way:
Here we will need to create a temporary variable that we use to store value temporarily
so that the other one gets empty and we can swap the values.
x=5
y=10
temp=x
x=y
y=temp
print(“The value of x after swapping is”,x)
print(“The value of y after swapping is”,y)
Now let me show you a simple trick to swap numbers in Python,
x = 5
y = 10
x, y = y, x
print(“The value of x after swapping is”,x)
print(“The value of y after swapping is”,y)
Wasn’t that simple?
2) Concatenating Strings
This is one of the useful and handy tricks. Let’s see how we can create a string from a list of characters. We will be using the join() method. Let’s check how it works,
characters = [‘p’, ‘y’, ‘t’, ‘h’, ‘o’, ‘n’]
word = “”.join(characters)
print(word)
Output:
Python
3) Reversing a String
Trust me reversing a string can’t be much simpler than this, Watch out…. We will use a simple concept of string slicing and negative indexing. We know that python can have a negative index, we just slice it and don’t provide any starting and ending value, just give the range of -1, i.e it will start from the last index till the first one, thus will reverse it. .
word = “orehgnimmargorp”
print(word[: : -1])
Output:
programming hero
Wasn’t that simple? Just simple slicing can get the work done.
4) Using ZIP with lists
Use the zip() function to combine several lists of the same length and print out the result.
language = [“python”, “java”, “c”]
creators = [“guido van rossum”, “james gosling”, “denis ricthie”]
for language, creators in zip(language, creators):
print(language, creators)
Output:
python guido van rossum
java james gosling
c denis ricthie
5) Converting lists into dictionary
This is one of the really useful tricks when you work on real projects on Django and Machine learning and more. Use zip() function like before, this time just invoke it in the dictionary constructor.
user = [“Peter”, “John”, “Sam”]
age = [23,19,34]
dictionary = dict(zip(user, age))
print(dictionary)
Output :
{“Peter” : 23, “John” : 23, “Sam” : 34}
6) The _ Operator
The _ operator might be something that you might not have heard of. Here _ is the output of the last executed expression. Let’s check how it works.
>>> 2+ 3
5
>>> _ # the _ operator, it will return the output of the last executed statement.
>>> 5
7) Opening a website
Python is all about cool stuff. Here is one of the coolest tricks: open a website using a python script. Sounds interesting?
import webbrowser
webbrowser.open(“https://www.programming-hero.com/”)
This is how we can open a website with just a single line of code.
8) Multiple user input
If you are thinking, it’s simple, just use two input functions and we are done. But I have another cooler way of doing it, first let’s check the old school way…
x = input(“Enter a number”)
y = input (“Enter another number”)
print(x)
print(y)
The cooler way,
x, y = input("Enter a two values: ").split()
print("value of x ", x)
print("value of y: ", y)
split() function helps in getting multiple inputs from the user . It breaks the given input by the specified separator. If a separator is not provided then any white space is a separator.
9) Colored Text
Bored with the old black and white console? This trick is to rescue you.
from termcolor import colored
print(colored(“Programming Hero”, “yellow”))
print(colored(“Programming Hero”, “red”))
Output :
Programming Hero
Programming Hero
10) The Walrus(:=) Operator
Walrus operators is one of the latest additions to Python. It was recently added to Python in Python version 3.8. It is an assignment expression that allows assignment directly in the expression.
Normal Way :
xs = [1,2,3]
n=len(xs)
if n>2:
print(n)
Here in this example, we declare a list and then declare a variable ‘n’ to assign the value of length of the list.
Using walrus operators,
xs = [1,2,3]
if (n:=len(xs)>2):
print(n)
Here, we declare and assign the value at the same time. That’s the power of the Walrus operator.
11) Shutting down a computer
Let’s check how we can shutdown a computer using just one line of code. For this we will use the OS module. It is one of the really important modules in python with many other functionalities.
import os
os.system(‘shutdown -s’)
That’s it!
12) List comprehension
List comprehension is an elegant way to define and create lists in python. We can create lists just like mathematical statements and in one line only. The syntax of list comprehension is easier to grasp. It’s a smart way to work with lists…
Normal Way:
Below code creates a list of odd square values,
odd_square = []
for x in range(1, 11):
if x % 2 == 1:
odd_square.append(x**2)
print (odd_square)
Using List Comprehension:
odd_square = [x ** 2 for x in range(1, 11) if x % 2 == 1]
(print odd_square)
We can have an expression, loop and condition in the same line. 5 lines of code in just 1 line. That’s List comprehension.
13) Multi-args Function:
It is one of the coolest Python tricks. Let’s say you are creating a function and you don’t know what will be the number of arguments that will be coming from the user side. Then how can we declare the parameters of the function? Here comes Multi-args functions, Check the below example that will add any number of values passed by the user.
def add(*num):
result=0
for i in num:
result=result+i
return result
print(add(1,2))
print(add(1,2,3,4,5))
Output:
3
15
Thus, multi-args function creates a list of parameters and then performs the operation.
14) Reading passwords as user input
Give users a great experience in simple menu based applications as well. Use the getpass module to read in passwords from the users in the console applications.
from getpass import getpass
username = input(‘username : ’)
password = getpass(‘password : ’ )
Output :
username : john23
password : *******
This hides the user input for the password field on the console.
15) Palindrome
I am sure you might have come across this problem. It is one of the university's favourite problems. Ever thought you could do that in just a single line of code? Check this out…
word = “wow”
palindrome = bool(word.find(word[: : -1]) + 1)
print(palindrome)
Output :
True
16) Fibonacci series
Oh, that’s another university’s favorite coding problem. And again I have a simple one line solution for you. Throw this code at your teacher and amaze him/her.
fibo = lambda n : n if n <= 1 else fibo(n-1) + fibo(n-2)
result = fibo(10)
print(result)
Wasn’t that simple?
17) Lambda Function
These are also called as anonymous functions because these functions don’t have names. These are rigorously used in Data Science, ML, Backend using Django and more. Let’s check an example to add two numbers.
Normal Function :
def add(a,b):
return a + b
add(2,3)
Lambda Function :
add = lambda a,b : a+b
add(2,3)
18) Measuring Execution Time
Want to test your program? Want to track the performance and the execution time? This feature is really gonna be helpful. Let’s check it out.
import time
startTime = time.time()
# your code
endTime = time.time()
totalTime = endTime - startTime
print("Execute code is= ", totalTime)
Output will be the milliseconds taken by the program to execute, Thus this will help to make the performance better and modify the code accordingly.
19) Removing duplicates from a List
Lists can have duplicate elements. But there are instances where we don’t want duplicate elements in the list. Let’s check how that can be done…
listNumbers = [20, 22, 24, 26, 28, 28, 20, 30, 24]
print("Original= ", listNumbers)
listNumbers = list(set(listNumbers))
print("After removing duplicate= ", listNumbers)
Output:
Original = [20, 22, 24, 26, 28, 28, 20, 30, 24]
After removing duplicate = [20, 22, 24, 26, 28, 30]
20) Manipulating Tuples
What’s that? How can we do that? We know tuples are immutable, then how can we manipulate it? We can! We are programmers and we can find ways for all problems. Check out below example,
tuple1=(1,2,3,4,5)
list1=list(tuple1)
list1.append(6)
tuple2=tuple(list1)
print(tuple2)
Output:
(1,2,3,4,5,6)
Simple? Just use list() and tuple() constructors and it’s done…
I hope these tricks will help you to get better at Python and it was super fun!
Want to learn Python is such a fun way? Try our app Programming Hero | Personalized Way To Learn Programming right now…